Running LLMs Locally on MacBook with ollama
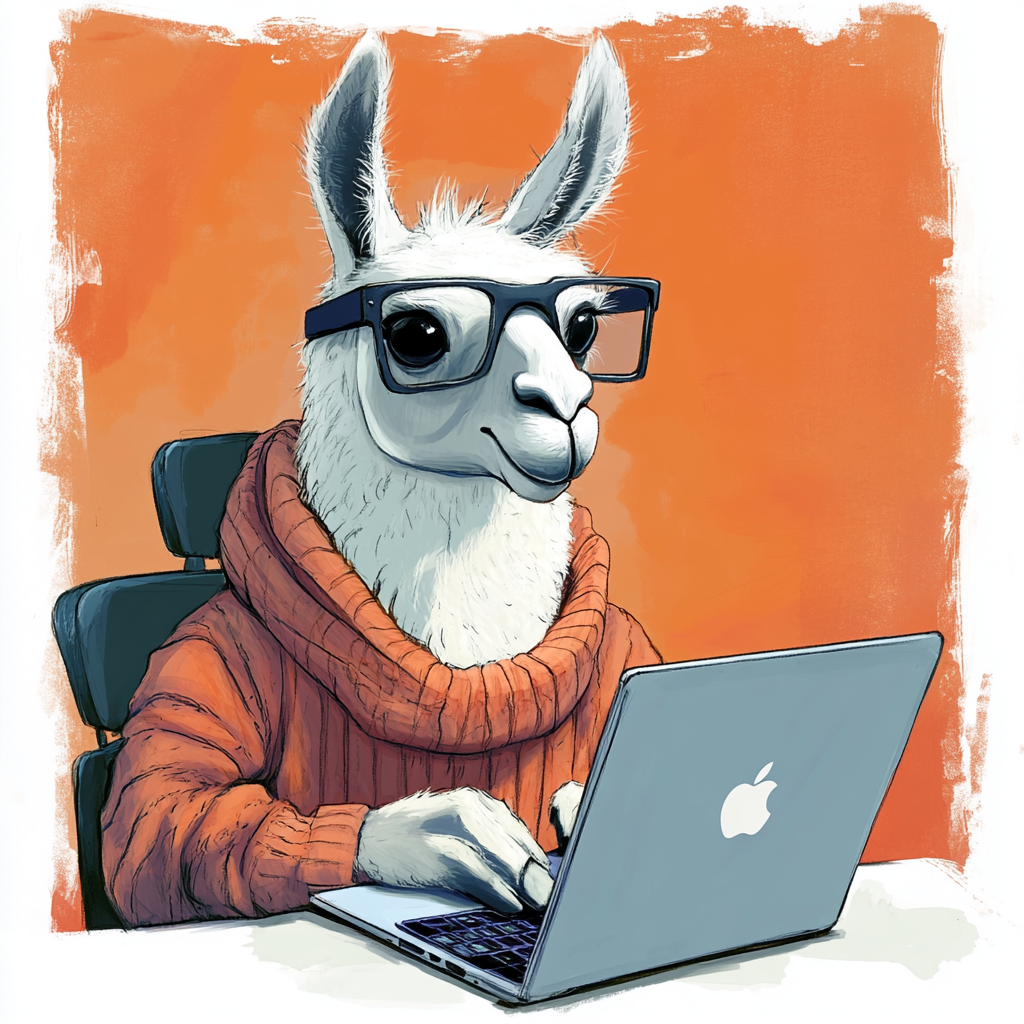
Thanks to recent advancements in AI and model optimization, it’s now possible to run powerful language models directly on your MacBook. This guide will walk you through the process of setting up and using Ollama, a tool that makes running LLMs locally both accessible and practical.
Understanding MacBook Capabilities and Model Selection
Before installing anything, it’s crucial to understand what your MacBook can handle. This section will help you make informed decisions about which models to use based on your available memory, ensuring smooth performance and preventing system slowdowns.
Memory Requirements and Quantization
Base Memory Requirements (Non-Quantized Models)
- 7B parameter models: ~16GB RAM
- 13B parameter models: ~32GB RAM
- 34B parameter models: ~64GB RAM
- 70B parameter models: ~128GB RAM
With Quantization (Q4_K_M or Q5_K_M recommended)
- 7B parameter models: ~8GB RAM
- 13B parameter models: ~16GB RAM
- 34B parameter models: ~32GB RAM
- 70B parameter models: ~64GB RAM
Note: While lower quantization levels exist (Q2, Q3), it’s recommended to stay with Q4 or higher for better output quality. Q4_K_M provides an excellent balance between memory usage and model performance.
Quick Reference Guide
Model Size | Minimum RAM (With Q4_K_M) | Recommended RAM | Notes |
---|---|---|---|
7B | 8GB | 16GB | Good starting point for most use cases |
13B | 16GB | 32GB | Better performance, more capabilities |
34B+ | 32GB | 64GB | Advanced use cases |
Remember: These requirements assume default context window sizes. Larger context windows will require additional memory.
Installation and Initial Setup
Setting up Ollama properly is the foundation for successfully running LLMs on your MacBook. Let’s walk through the installation process and initial configuration to ensure everything works correctly.
Basic Installation
For MacBook:
- Download Ollama from https://ollama.com/download
- Unzip the downloaded file
- Drag the Ollama app to your Applications folder
- Launch Ollama from your Applications folder
- You’ll see the Ollama icon appear in your menu bar
After installation, open Terminal and verify it’s working:
ollama --version
Environment Configuration
Customizing where Ollama stores its data and how it logs information can help you better manage your system resources and troubleshoot issues when they arise.
# Set custom model directory (optional)
export OLLAMA_MODELS="/path/to/your/models"
# Enable debug logging (if needed)
export OLLAMA_DEBUG=1
First-Time Setup Verification
# Check if Ollama service is running
lsof -i :11434
# View Ollama logs
cat ~/.ollama/logs/server.log
Working with Models
Once Ollama is installed, you’ll need to know how to manage and interact with different models. This section covers the essential commands and best practices for working with models effectively.
Pulling and Managing Models
# Pull a specific model
ollama pull llama3.2
# List available models
ollama list
# Remove a model
ollama rm modelname
# Show model information
ollama show modelname
Interactive Use
# Start an interactive session
ollama run llama3.2
# Available commands in interactive mode
/help # Show help message
/list # List available models
/model # Show current model info
/system # Set system message
/template # Set custom template
/quit # Exit the session
Example Interactions
> Write a Python function to calculate the Fibonacci sequence
Here's a Python function to calculate the Fibonacci sequence:
def fibonacci(n):
if n <= 0:
return []
elif n == 1:
return [0]
sequence = [0, 1]
while len(sequence) < n:
sequence.append(sequence[-1] + sequence[-2])
return sequence
# Example usage:
print(fibonacci(10)) # [0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
Performance Optimization
Running LLMs locally can be resource-intensive. Understanding how to optimize performance will help you get the most out of your MacBook while preventing overload.
Memory Management
-
Monitor Resource Usage While you can use
top
to monitor system resources, a much better tool for Apple Silicon Macs is asitop. It provides a beautiful, real-time view of your CPU, GPU, and Neural Engine usage:# Install asitop brew install asitop # Run monitoring sudo asitop
This will show you a clean, detailed view of how your MacBook is handling the LLM workload, including Metal GPU usage.
-
Optimize Model Loading
# Pre-load model ollama run llama3.2 "" # Set keep-alive duration export OLLAMA_KEEP_ALIVE="30m"
-
Context Window Management The context window determines how much previous text the model can “remember.” You can set this in two ways:
a. In your Modelfile:
FROM llama3.2 PARAMETER num_ctx 2048
b. When making API calls:
client.generate( prompt="Your prompt here", options={"num_ctx": 2048} )
Note: Larger context windows require more memory. For example:
- 2K tokens ≈ baseline memory usage
- 4K tokens ≈ 2x baseline memory
- 8K tokens ≈ 4x baseline memory
GPU Acceleration
Ollama automatically uses Metal API on Apple Silicon Macs. Verify GPU usage:
# Check if Metal is being used
cat ~/.ollama/logs/server.log | grep "metal"
API Integration
One of Ollama’s strongest features is its API, which allows you to integrate LLMs into your applications. Here’s how to interact with Ollama programmatically using Python and HTTPX, a modern HTTP client.
Python API Example with Chat Support
import httpx
import asyncio
from typing import List, Dict, Any
from datetime import datetime
class OllamaAPI:
def __init__(self, base_url: str = "http://localhost:11434"):
self.base_url = base_url
self.client = httpx.AsyncClient(timeout=30.0) # Increased timeout for larger responses
async def generate(self, prompt: str, model: str = "llama3.2",
system: str = None) -> Dict[str, Any]:
"""Simple generation without chat history"""
messages = []
if system:
messages.append({"role": "system", "content": system})
messages.append({"role": "user", "content": prompt})
response = await self.client.post(
f"{self.base_url}/api/chat",
json={
"model": model,
"messages": messages,
"stream": False
}
)
return response.json()
async def chat(self, messages: List[Dict[str, str]],
model: str = "llama3.2") -> Dict[str, Any]:
"""Chat with history support"""
response = await self.client.post(
f"{self.base_url}/api/chat",
json={
"model": model,
"messages": messages,
"stream": False
}
)
return response.json()
async def close(self):
await self.client.aclose()
async def main():
api = OllamaAPI()
try:
# Example 1: Simple generation with system prompt
response = await api.generate(
"Explain what makes Python great for data science",
system="You are a helpful programming instructor"
)
print("Example 1: Simple Generation")
print(response['message']['content'])
print("\n" + "="*50 + "\n")
# Example 2: Multi-turn conversation
conversation = [
{"role": "system", "content": "You are a Python expert. Be concise."},
{"role": "user", "content": "How do I read a CSV file?"},
{"role": "assistant", "content": "Use pandas: `df = pd.read_csv('file.csv')`"},
{"role": "user", "content": "Now how do I filter rows?"}
]
response = await api.chat(conversation)
print("Example 2: Chat Conversation")
print(response['message']['content'])
print("\n" + "="*50 + "\n")
# Example 3: Chain of thought reasoning
cot_prompt = [
{"role": "system", "content": "You solve problems step by step, showing your reasoning."},
{"role": "user", "content": """
Solve this problem:
A shop keeper bought 100 items at $2 each.
They sold 80% of items at $3 each.
The rest were damaged and sold at 50% loss.
Calculate the total profit or loss.
"""}
]
response = await api.chat(cot_prompt)
print("Example 3: Chain of Thought Reasoning")
print(response['message']['content'])
finally:
await api.close()
if __name__ == "__main__":
asyncio.run(main())
Advanced Customization with Modelfiles
Modelfiles allow you to customize how models behave and respond. Here are some examples of specialized models for different purposes.
Chain of Thought Reasoning Model
This model is designed to break down problems step by step:
FROM llama3.2
# Set lower temperature for more focused responses
PARAMETER temperature 0.3
PARAMETER num_ctx 4096
# Configure the model to think step by step
SYSTEM """You are a methodical problem solver. For each problem:
1. Break it down into smaller parts
2. Solve each part step by step
3. Show your reasoning clearly
4. Verify your solution
Always start with 'Let's solve this step by step:'"""
Math Problem Solver
This model uses a specific template to solve math problems systematically:
FROM llama3.2
PARAMETER temperature 0.1
PARAMETER num_ctx 2048
SYSTEM """You are a math teacher who solves problems by:
1. Understanding the given information
2. Planning the solution
3. Executing step by step
4. Checking the answer"""
TEMPLATE """
PROBLEM: {{ .Prompt }}
Let's solve this:
1) First, let's understand what we know:
2) Here's how we'll solve it:
3) Solution steps:
4) Final answer:
5) Verification:
"""
Interview Assistant Model
This model helps prepare for technical interviews:
FROM llama3.2
PARAMETER temperature 0.7
PARAMETER num_ctx 4096
SYSTEM """You are an expert interview coach specializing in technical interviews.
For each question:
1. First, ask clarifying questions
2. Discuss potential approaches
3. Provide a solution
4. Mention time/space complexity
5. Suggest follow-up questions"""
Create and use these models with:
# Create the model
ollama create math-solver -f Modelfile
# Use the model
ollama run math-solver "Solve: If a train travels 120 km in 2 hours, what's the average speed?"
Best Practices and Tips
Learning from common patterns and pitfalls can save you time and resources. These best practices have been collected from real-world usage and community feedback.
Memory Management
- Start with smaller models and test performance
- Monitor memory usage with Activity Monitor
- Close unnecessary applications
- Use quantized models when possible
- Consider context window size impact
Performance
- Pre-load frequently used models
- Use appropriate temperature settings
- Implement proper error handling in applications
- Consider batch processing for large tasks
Development
- Start with API testing tools (like Postman)
- Implement proper timeout handling
- Use async/await for better performance
- Implement proper error handling
- Consider implementing retry logic
Troubleshooting Common Issues
Even with careful setup, you might encounter issues. This section helps you identify and resolve common problems quickly, getting you back to productive work with your models.
Common Problems and Solutions
-
Model Loading Issues
# Check logs cat ~/.ollama/logs/server.log # Verify model is downloaded ollama list
-
Memory Problems
- Reduce context window size
- Use quantized models
- Clear model cache
rm -rf ~/.ollama/models/*
-
API Connection Issues
- Verify Ollama is running
- Check port availability
- Review firewall settings
Conclusion
Running LLMs locally with Ollama provides a powerful and flexible way to work with AI models while maintaining privacy and control. By understanding your hardware capabilities, choosing appropriate models, and following best practices, you can create efficient and effective AI-powered applications.
Remember to:
- Start with smaller models and gradually scale up
- Monitor system resources
- Use appropriate quantization and optimization
- Implement proper error handling
- Stay updated with Ollama’s latest features and improvements
The field of LLMs is rapidly evolving, and Ollama continues to improve its capabilities. Keep an eye on the official documentation and community resources for the latest updates and best practices.
Happy coding with your local LLMs!